What is AWS Cognito?
AWS Cognito is a fully managed service that helps developers manage user authentication and identity in applications. It allows you to add sign-up, sign-in, and access control to your applications quickly and securely, without having to build these features from scratch.
At its core, AWS Cognito provides an easy way to authenticate users, store user data, and synchronize that data across devices. It also integrates with other AWS services, making it an ideal solution for developers looking to build secure and scalable applications in the cloud. Whether you're building a web app, mobile app, or even an IoT system, Cognito enables you to easily authenticate and manage your users.
Why it's essential:
- Scalability: Cognito is designed to scale effortlessly, handling millions of users and authentication requests without additional infrastructure.
- Security: With built-in features like encryption, multi-factor authentication (MFA), and compliance with standards like GDPR and HIPAA, AWS Cognito ensures user data is protected.
- Integration: It integrates smoothly with other AWS services, such as AWS Lambda, API Gateway, and AWS IAM, allowing you to build secure, serverless applications.
Key Benefits of AWS Cognito
AWS Cognito simplifies and accelerates the process of building secure, scalable user authentication systems. Here’s how:
- User Management Simplified:
Cognito allows you to easily create and manage user pools (repositories of users) and configure user attributes like email, phone number, or custom data. - Federated Identity Support:
With Cognito, you can authenticate users from social identity providers (Google, Facebook, Amazon) or enterprise identity providers (Active Directory, SAML). This enables seamless single sign-on (SSO) and external identity integration. - Security Built-In:
Cognito handles user authentication, password policies, and security protocols like MFA out of the box. It also helps ensure compliance with various regulatory standards. - Scalable Infrastructure:
As your app grows, Cognito scales with it. Whether you're handling thousands or millions of users, you don't need to worry about scaling your authentication infrastructure. - Easy Integration with AWS Services:
AWS Cognito integrates seamlessly with other AWS services like AWS Lambda for custom workflows, Amazon API Gateway for secure API access, and AWS IAM for managing user permissions.
When to Use AWS Cognito?
AWS Cognito is an ideal solution for a wide variety of applications, ranging from small startups to large enterprise solutions. Here are some common use cases:
- Small Applications and Startups:
For small projects and startups, implementing user authentication and management without Cognito would require significant custom coding and server management. AWS Cognito simplifies the process, handling everything from user registration to password management, while you focus on building your application. - Mobile and Web Apps:
For both mobile and web applications, Cognito provides an easy-to-use authentication system, offering native SDKs for quick integration with your apps. Whether your app is for social media, online banking, or e-commerce, Cognito provides secure and reliable user authentication. - Enterprise-Level Solutions:
For larger organizations, Cognito can integrate with corporate identity providers like Active Directory and SAML. This allows for enterprise-grade features like Single Sign-On (SSO) and multi-factor authentication (MFA), all within a scalable, secure platform. - Serverless Architectures:
AWS Cognito is a perfect match for serverless applications, as it works seamlessly with AWS Lambda and API Gateway. This reduces the need for managing dedicated servers while providing a secure and scalable user management solution.
Core Features of AWS Cognito
User Pools and Identity Pools
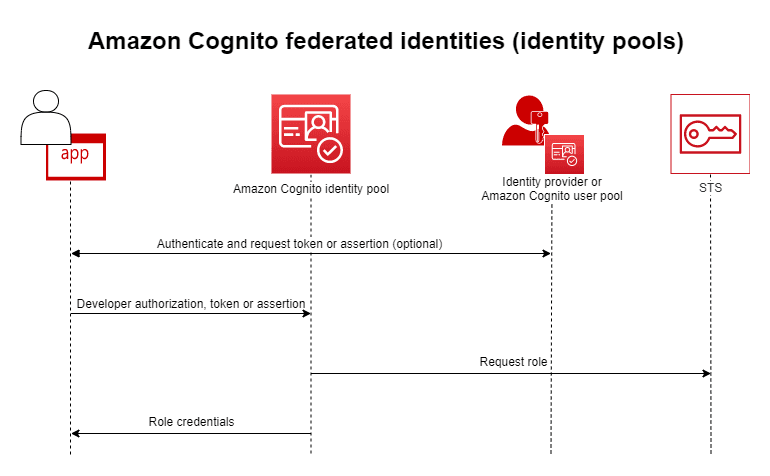
Source: AWS Documentation
At the heart of AWS Cognito are User Pools and Identity Pools. These two components help manage user authentication and authorization.
- User Pools:
A User Pool is a directory of users where you can manage sign-up, sign-in, and profile information. You can customize it with user attributes (like name, email, phone number) and configure security features such as password policies, account recovery, and multi-factor authentication (MFA). User Pools are primarily used for handling the authentication process. - Identity Pools:
Identity Pools are used for enabling users to access AWS resources securely. While User Pools handle the authentication, Identity Pools provide federated identities that allow authenticated users to access AWS services. For instance, once a user is authenticated via a User Pool, the Identity Pool assigns a set of AWS credentials for secure access to other AWS resources.
Together, these pools enable a smooth and secure authentication and authorization experience for your applications, whether you're building a simple app or a large enterprise system.
Authentication Flow in AWS Cognito
Understanding how AWS Cognito manages user authentication is key to leveraging its full potential. The process is broken down into a series of steps:
- Sign-Up: The user creates an account, providing necessary information such as email, username, and password. AWS Cognito can also support sign-ups using social identity providers (like Facebook or Google).
- Sign-In: After signing up, the user logs in using their credentials (username/password or third-party authentication). Cognito validates the credentials and generates authentication tokens (ID token, access token, refresh token).
- Token Management: Cognito issues three types of tokens:
- ID Token: Contains user information and is used to authenticate the user to your app.
- Access Token: Used to authorize requests to AWS services and APIs.
- Refresh Token: Used to obtain new tokens without requiring the user to log in again.
Cognito handles token expiration and refresh automatically, ensuring a seamless user experience.
creating a user via the AWS SDK for Cognito.
javascript const cognito = new AWS.CognitoIdentityServiceProvider(); const params = { ClientId: 'your_client_id', Password: 'user_password', Username: 'user_name' }; cognito.adminCreateUser(params, function(err, data) { if (err) console.log(err, err.stack); else console.log(data); }); |
This code creates a new user in your Cognito User Pool, initializing the registration process.
Multi-Factor Authentication (MFA) Setup
Multi-factor authentication (MFA) is an important feature for securing user accounts. With Cognito, enabling MFA is straightforward. MFA adds an additional layer of security by requiring the user to provide a second form of verification (such as a code sent to their phone) in addition to their password.
To enable MFA, you can configure it via the AWS Console or programmatically through the AWS SDK. This provides greater security for your application, ensuring that even if a password is compromised, an attacker cannot access the account without the second authentication factor.
Authentication & Token Management
Source: AWS Documentation
The Role of Tokens in Cognito Authentication
Tokens play a crucial role in AWS Cognito’s authentication mechanism. They help maintain the session and provide secure access to resources.
- ID Token:
This token contains claims about the user, such as their username, email, and other custom attributes. It is used to authenticate the user and verify their identity within your app. - Access Token:
The access token is used to authorize the user to access specific AWS resources or APIs. It’s often passed as a bearer token in HTTP headers when making requests. - Refresh Token:
The refresh token allows users to renew their authentication tokens without needing to log in again. This is especially useful for long-running sessions and helps avoid frequent logins.
Understanding how these tokens work and how they are validated is key for securing your applications.
Example of decoding and validating JWT tokens.
javascript import jwt from 'jsonwebtoken'; const decodedToken = jwt.decode(idToken, { complete: true }); console.log(decodedToken); |
This code decodes a JWT token and logs the contents, which can be useful for verifying the token and extracting user information.
Managing Sessions with Cognito
AWS Cognito takes care of session management by handling token expiration and renewal. Once a user signs in, Cognito issues an access token and an ID token, both of which are valid for a limited period (usually 1 hour). The refresh token is valid for a longer period (usually 30 days or more) and can be used to obtain new access and ID tokens when they expire.
When the user’s tokens expire, Cognito automatically uses the refresh token to obtain new tokens, ensuring the user remains authenticated without having to re-enter credentials.
Integration of AWS Cognito with Applications
Integrating Cognito with Web and Mobile Apps
Integrating AWS Cognito with your web or mobile application is straightforward, thanks to the SDKs AWS provides for various platforms (JavaScript, iOS, Android, and more). These SDKs allow you to add authentication and user management to your app with minimal code.
Here’s how you can integrate Cognito into a web or mobile app:
1. Set up your User Pool:
First, you need to create a User Pool in AWS Cognito. This pool will store your user profiles and handle the user sign-up and sign-in process.
2. Install AWS Amplify SDK:
AWS Amplify is a framework that simplifies the integration of AWS services, including Cognito, into web and mobile apps. It provides a set of libraries to handle user authentication, API calls, and more.
Example for Web (JavaScript):
bash npm install aws-amplify |
In your JavaScript code, you can then configure AWS Amplify to connect to your Cognito User Pool:
javascript import Amplify from 'aws-amplify'; import awsconfig from './aws-exports'; // Config file created by Amplify CLI Amplify.configure(awsconfig); |
3. Sign-Up and Sign-In:
Once AWS Amplify is configured, adding user sign-up and sign-in is a breeze. Here’s how you can implement it:
javascript import { Auth } from 'aws-amplify'; // Sign-Up async function signUp() { try { const user = await Auth.signUp({ username: 'testUser', password: 'TestPassword123', attributes: { email: '[email protected]' }, }); console.log(user); } catch (error) { console.error('Error signing up:', error); } } // Sign-In async function signIn() { try { const user = await Auth.signIn('testUser', 'TestPassword123'); console.log('Logged in:', user); } catch (error) { console.error('Error signing in:', error); } } |
This code allows users to sign up and sign in to your application using AWS Cognito with just a few lines of code.
API Security with Cognito
AWS Cognito also helps you secure your APIs by managing user authentication and authorization. After users authenticate through Cognito, you can use AWS IAM roles and policies to control access to your backend resources, such as APIs exposed via Amazon API Gateway.
Securing an API Gateway with Cognito Authentication:
To secure your API, you need to configure your API Gateway to use Cognito as an authorizer. Here’s an example IAM policy to control access to your API Gateway, allowing only authenticated users to access certain resources.
Example IAM policy for securing API Gateway with Cognito authentication.
json { "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Action": "execute-api:Invoke", "Resource": "arn:aws:execute-api:us-east-1:123456789012:abcd1234/*/GET/your-api" } ] } |
This policy ensures that only requests authenticated by Cognito (with valid tokens) can access the API endpoint.
You can also configure Cognito to enforce policies based on different user roles or permissions, adding an additional layer of security to your application.
Federation and External Identity Providers
Federating identities through AWS Cognito is a powerful feature that allows you to authenticate users from external identity providers like Google, Facebook, or corporate identity systems such as Active Directory or SAML. This is often referred to as Federated Authentication.
Here’s how to enable external identity providers:
- Set Up Social Identity Providers (e.g., Google, Facebook):
AWS Cognito allows you to easily integrate with popular social providers. After configuring the provider in the Cognito console, users can log in using their existing social media accounts. - Configure Enterprise Identity Providers (e.g., SAML, Active Directory):
For enterprise applications, Cognito supports integration with SAML-based identity providers, enabling Single Sign-On (SSO) for corporate users.
By integrating external identity providers, you can make your app more user-friendly and increase adoption by allowing users to log in with the accounts they already use.
Advanced Features and Customization
Custom User Attributes and Lambda Triggers
AWS Cognito is highly customizable. You can extend the functionality of Cognito by using Lambda triggers to run custom workflows during various stages of the authentication process.
For example, you can add custom user attributes (e.g., user roles, preferences) during the sign-up process or trigger an event every time a user logs in.
Example Lambda trigger to add custom user attributes.
javascript exports.handler = async (event) => { |
This Lambda function adds a custom attribute (custom:role) to the user's profile during the sign-up process, making it possible to assign roles dynamically.
Advanced Use Cases for Lambda Triggers:
- Custom validation of user attributes (e.g., check if the email is valid).
- Sending notifications or performing background tasks (e.g., sending a welcome email).
- Modifying user attributes after authentication (e.g., upgrading the user role based on their subscription).
Advanced Security Features
AWS Cognito also offers several advanced security features to fine-tune your app’s authentication process. Some of these include:
- Custom Password Policies:
You can configure complex password policies to enforce stronger security, such as minimum password length, required character types, and password expiration rules. - Multi-Factor Authentication (MFA):
MFA adds an extra layer of security by requiring users to provide a second form of authentication (like a code sent to their mobile device) in addition to their password. - Custom Policies:
Cognito supports the creation of custom security policies for access control, allowing you to restrict or allow certain actions based on user attributes or roles.
These features help ensure that your application is secure and compliant with security best practices.
Real-World Use Cases
Example 1: Basic Authentication for a Simple App
For a simple app (e.g., a to-do list app), you might just need basic authentication for users to sign up, log in, and access their personal data. Here’s a quick rundown on how Cognito can be used for this:
- Create a Cognito User Pool:
Set up a User Pool in Cognito to manage users and their sign-up/sign-in processes. - Add Authentication to the App:
Use AWS Amplify or the Cognito SDK to implement authentication in your app. - Access User Data:
After logging in, users can securely access their personal data stored in DynamoDB or another AWS service.
By using Cognito, you avoid having to handle user data directly, ensuring that sensitive information is managed securely by AWS.
Example 2: Scalable and Secure Authentication in Serverless Apps
For more complex applications, particularly those using serverless architectures, AWS Cognito integrates seamlessly with services like AWS Lambda and API Gateway to provide authentication and authorization.
Here’s how it works in a serverless app:
- User Authentication via Cognito:
Cognito handles the user authentication and issues tokens. - Securing API Gateway:
Use the Cognito tokens to secure API Gateway endpoints. Only authenticated users can invoke these APIs. - Serverless Lambda Functions:
Lambda functions can be used to process requests, interact with DynamoDB or other AWS services, and return results to the user.
This combination allows you to build highly scalable and secure applications without managing traditional infrastructure.
Troubleshooting and Debugging
Common Issues and Their Solutions
When working with AWS Cognito, developers and administrators may face common challenges. Here’s a breakdown of frequent issues and how to resolve them:
- Token Expiration:
- Issue: Cognito tokens (ID, Access, and Refresh tokens) have a limited lifespan. When they expire, users may lose access to protected resources.
- Solution: Implement automatic token refreshing using Cognito’s refreshToken method. This allows you to obtain a new ID and Access token without requiring the user to log in again.
Example: Refreshing tokens with AWS Amplify:
javascript import { Auth } from 'aws-amplify'; async function refreshToken() { try { const currentUser = await Auth.currentAuthenticatedUser(); const newSession = await Auth.currentSession(); console.log('New Access Token:', newSession.getAccessToken().getJwtToken()); } catch (error) { console.error('Error refreshing token:', error); } } |
- MFA Issues:
- Issue: Users may face issues when setting up or using Multi-Factor Authentication (MFA), especially if they don’t receive the MFA code or the code doesn’t work.
- Solution: Ensure that users' phone numbers or email addresses are correctly configured in Cognito. If users don’t receive MFA codes, check if SMS or email delivery is properly configured in the AWS region.
- API Integration Problems:
- Issue: API calls that require authentication may fail if the token isn’t passed correctly or if the API Gateway isn’t properly configured to use Cognito as the authorizer.
- Solution: Double-check your API Gateway's authorizer configuration to ensure it’s set to use the correct Cognito User Pool. Also, verify that tokens are sent correctly in the Authorization header.
Example of adding token to API requests:
javascript import axios from 'axios'; async function fetchData() { const token = await Auth.currentSession(); const idToken = token.getIdToken().getJwtToken(); axios.get('https://your-api-endpoint.com/data', { headers: { Authorization: `Bearer ${idToken}` } }).then(response => { console.log(response.data); }).catch(error => { console.error('API call failed:', error); }); } |
Advanced Troubleshooting for Large-Scale Deployments
When managing large-scale or enterprise applications with Cognito, issues can become more complex due to factors like high traffic, multiple identity providers, and integrations with numerous AWS services. Here are some advanced strategies:
- Check Cognito Logs:
Use Amazon CloudWatch Logs to monitor and debug user pool activity, including failed sign-ins, errors, and unusual behavior. You can configure CloudWatch to capture Lambda function logs triggered by Cognito events (e.g., user registration). - Optimize for Scalability:
If your application experiences high user volumes, ensure that you are using Auto Scaling for your Cognito User Pools and are following best practices for scaling Lambda functions and API Gateway. Be aware of throttling limits and configure your architecture to handle high concurrency. - User Pool Configuration Review:
For large-scale applications, reviewing the configurations of user pools is essential. Ensure that your pool has the right policies (password strength, MFA) and that your integrations with federated identity providers (Google, Facebook, SAML) are functioning as expected. - Examine Custom Lambda Triggers:
Advanced customizations through Lambda triggers can sometimes introduce errors. Be sure to thoroughly test these functions and implement logging within Lambda to debug unexpected behaviors during the sign-up, sign-in, or post-authentication flows.
Security Best Practices
Securing User Data and Privacy
Protecting user data is critical, and AWS Cognito provides several mechanisms to ensure security:
- Encryption in Transit and at Rest:
- In Transit: AWS Cognito uses SSL/TLS encryption to ensure that all data transmitted between users and Cognito (e.g., during login or token exchanges) is secure.
- At Rest: User data stored in Cognito (such as user attributes and metadata) is encrypted using AWS KMS (Key Management Service).
- Best Practices:
- Use HTTPS for all API requests and secure your web applications using SSL certificates.
- Enable encryption for all stored data, including custom attributes or sensitive information, using AWS encryption options.
- Implement Multi-Factor Authentication (MFA): MFA adds an extra layer of security by requiring users to verify their identity with a second factor, such as a one-time passcode (OTP) sent via SMS or email. Enforcing MFA in Cognito ensures that even if an attacker obtains a user’s password, they cannot access the account without the second factor.
To enable MFA:- Go to your Cognito User Pool settings and enable MFA options like SMS-based OTP or Time-Based One-Time Password (TOTP) (e.g., via Google Authenticator).
Use Fine-Grained Access Controls: Implement role-based access control (RBAC) using IAM roles. Ensure that different users or groups in Cognito are assigned the appropriate IAM roles, and use these roles to restrict access to specific resources (e.g., APIs or data stored in DynamoDB).
Example IAM Policy to restrict access:
json { "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Action": "dynamodb:GetItem", "Resource": "arn:aws:dynamodb:us-east-1:123456789012:table/UserData" } ] } |
Compliance and Enterprise Security
AWS Cognito helps organizations meet compliance requirements for various industry standards, including:
- GDPR (General Data Protection Regulation): AWS Cognito can help you comply with GDPR by providing controls to manage user data retention, data access requests, and the ability to delete user data when needed.
- HIPAA (Health Insurance Portability and Accountability Act): Cognito is HIPAA-eligible, allowing healthcare providers and organizations in the medical sector to store and manage health-related data securely.
- SOC 2 and ISO Certifications: AWS Cognito is compliant with SOC 2 and ISO 27001, ensuring that user authentication and data management practices meet stringent security standards.
Best Practices for Enterprise Security:
- Use custom security policies in Cognito to enforce stricter controls over access to your resources.
- Review the Audit Logs in CloudTrail to track and monitor user activities.
- Regularly rotate keys for AWS services and enable continuous monitoring with CloudWatch and GuardDuty.
Pricing and Cost Optimization
AWS Cognito Pricing Overview
Understanding the pricing structure of AWS Cognito is essential for managing costs effectively. The pricing is based on two primary components:
- Monthly Active Users (MAUs):
- Cognito charges are based on the number of MAUs, which refers to users who authenticate in a given month.
- Free Tier: AWS offers a free tier for up to 50,000 MAUs per month. After that, you are charged per MAU, with rates varying based on the region.
- Multi-Factor Authentication (MFA):
- SMS-based MFA incurs an additional cost, which depends on the country where the message is sent.
- TOTP-based MFA (using apps like Google Authenticator) is free of charge.
- Other Features:
- Federated Identities: When integrating with third-party identity providers (e.g., Facebook, Google, or SAML), you may incur additional costs.
- Custom Authentication Flows: Using AWS Lambda triggers with Cognito may incur additional Lambda invocation charges.
Here’s a simplified breakdown of the pricing structure:
Service | Pricing |
Monthly Active Users (MAUs) | Free for up to 50,000, then $0.0055 per MAU |
MFA (SMS) | $0.075 per SMS message sent (varies by country) |
MFA (TOTP) | Free (via authenticator apps like Google Authenticator) |
Federated Identities | Free for up to 50,000 users, then $0.00325 per MAU |
Cost Optimization Tips for Cognito
- Limit SMS-based MFA usage:
Use TOTP MFA instead of SMS-based MFA whenever possible to avoid extra costs. - Consolidate User Pools:
Instead of creating multiple user pools for different applications, try to consolidate users across apps to minimize MAU counts and keep costs down. - Leverage Free Tier:
Take full advantage of Cognito’s free tier by limiting the number of active users or using custom authentication mechanisms that don’t incur extra costs. - Monitor Usage:
Regularly monitor the number of MAUs through the AWS Console or use Amazon CloudWatch to keep track of usage patterns and anticipate any spikes in costs.
Alternatives to AWS Cognito
Comparison with Firebase Authentication, Auth0, and Okta
AWS Cognito is a powerful identity and authentication solution, but it’s not the only option. Let’s compare it with other popular authentication providers to help you choose the best solution for your app:
Feature | AWS Cognito | Firebase Authentication | Auth0 | Okta |
Primary Focus | Identity and access management for web and mobile | Simple authentication for web and mobile apps | Flexible identity management and authentication | Enterprise-grade identity management and security |
Federation Support | Social logins, SAML, OIDC | Google, Facebook, Twitter | Extensive (Google, Facebook, enterprise providers) | Extensive (Active Directory, SAML, LDAP) |
Security Features | MFA, IAM roles, encryption at rest and in transit | MFA (TOTP, SMS), encryption at rest | MFA, role-based access, enterprise security | MFA, adaptive authentication, enterprise security |
Customization | High (Lambda triggers, custom flows) | Limited customization | High (custom workflows, roles) | High (custom enterprise workflows) |
Pricing | Free tier for up to 50,000 MAUs, pay-as-you-go | Free tier for up to 10K verifications, pay-as-you-go | Free for 7K MAUs, pay-as-you-go | Enterprise pricing |
Scalability | Excellent for both small and large apps | Good for small to medium apps | Excellent scalability | Ideal for large enterprise solutions |
Pros and Cons:
Service | Pros | Cons |
AWS Cognito | - Seamless AWS integration - Cost-effective for small to medium apps - Strong federated identity support (e.g., Google, Facebook, SAML) | - Complex setup for beginners - Limited pre-built UI components |
Firebase Authentication | - Quick and easy setup for simple apps - Smooth integration with Firebase services | - Limited enterprise features - Fewer customization options |
Auth0 | - Highly customizable and flexible - Excellent for SaaS apps - Extensive third-party integrations | - Expensive at scale - More complex setup compared to Firebase or Cognito |
Okta | - Enterprise-grade security and compliance - Advanced authentication policies - Great for large-scale deployments | - High cost, especially for smaller apps - Overkill for basic use cases |
Choosing the Right Authentication Solution
To choose the right solution for your app:
- For small to medium apps that need a cost-effective, easy-to-implement solution, Firebase Authentication or Cognito is ideal.
- For enterprise-grade solutions with complex security needs and deep integration, Auth0 or Okta may be better suited.
Conclusion
Key Takeaways
AWS Cognito offers a scalable, secure identity management solution with features like user pools, MFA, Lambda triggers, and federated identity support. It integrates well with AWS services, making it a great choice for both small apps and enterprise-level applications. Some key points to remember:
- Cognito is ideal for users already leveraging AWS services.
- Offers a free tier for up to 50,000 MAUs, making it a cost-effective solution for startups and small businesses.
- Advanced security features like MFA, encryption, and customizable user flows provide robust protection.
Future of Authentication and Identity Management
The future of authentication is shifting towards passwordless login and decentralized identity systems. As technologies like WebAuthn and blockchain-based identities evolve, the landscape of identity management will continue to change. Expect more advanced AI-driven authentication methods for better security and user experience.